1. 프로젝트 생성
django-admin startproject UploadProject
2. APP 생성
django-admin startapp ajaxfilesupload
3. Settings.py 수정
[APP 등록]
INSTALLED_APPS = [
...
''ajaxfilesupload.apps.AjaxfilesuploadConfig',',
]
[template 설정]
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [os.path.join(BASE_DIR, 'templates')],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
4. template 및 html 생성
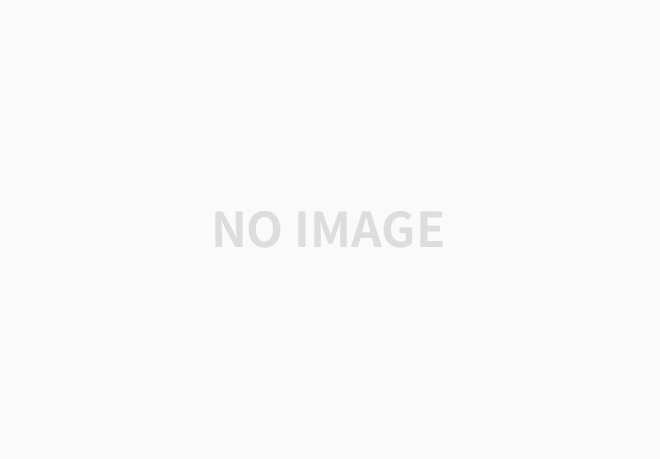
[files_upload.html]
<!DOCTYPE html>
<html>
<head>
<title>Django AJAX Files Upload</title>
</head>
<body>
<div style="width: 500px; margin: auto;">
<fieldset name="Multiple Files Upload">
{% if msg %} {% autoescape off %} {{ msg }} {% endautoescape %} {% endif %}
<div id="msg"></div>
<p>
{% csrf_token %}
<input type="file" id="multiFiles" name="files[]" multiple="multiple"/>
<button id="upload">Upload</button>
</p>
</fieldset>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js" crossorigin="anonymous"></script>
<script type="text/javascript">
$(document).ready(function (e) {
$('#upload').on('click', function () {
var form_data = new FormData();
var ins = document.getElementById('multiFiles').files.length;
if(ins == 0) {
$('#msg').html('<span style="color:red">Select at least one file</span>');
return;
}
for (var x = 0; x < ins; x++) {
form_data.append("files[]", document.getElementById('multiFiles').files[x]);
}
csrf_token = $('input[name="csrfmiddlewaretoken"]').val();
//console.log(csrf_token);
form_data.append("csrfmiddlewaretoken", csrf_token);
$.ajax({
url: 'http://IP:PORT', // point to server-side URL
dataType: 'json', // what to expect back from server
cache: false,
contentType: false,
processData: false,
//data: {'data': form_data, 'csrfmiddlewaretoken': csrf_token},
data: form_data,
type: 'post',
success: function (response) { // display success response
$('#msg').html(response.msg);
},
error: function (response) {
$('#msg').html(response.message); // display error response
}
});
});
});
</script>
</body>
</html>
5. UploadProject의 urls.py 설정
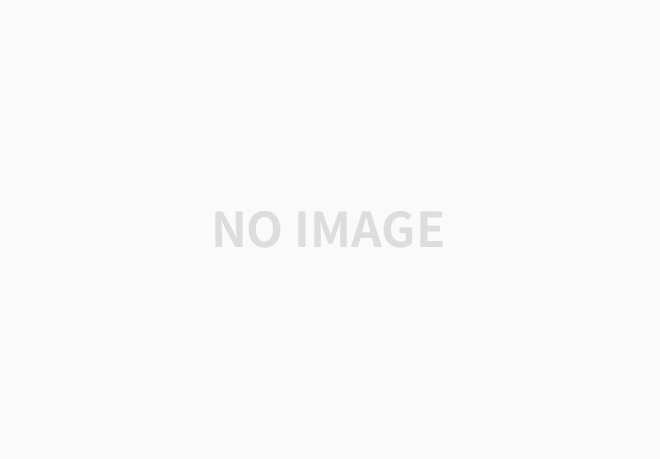
[urls.py]
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('', include('ajaxfilesupload.urls')),
path('admin/', admin.site.urls),
]
6. ajaxfilesupload APP의 urls.py 설정
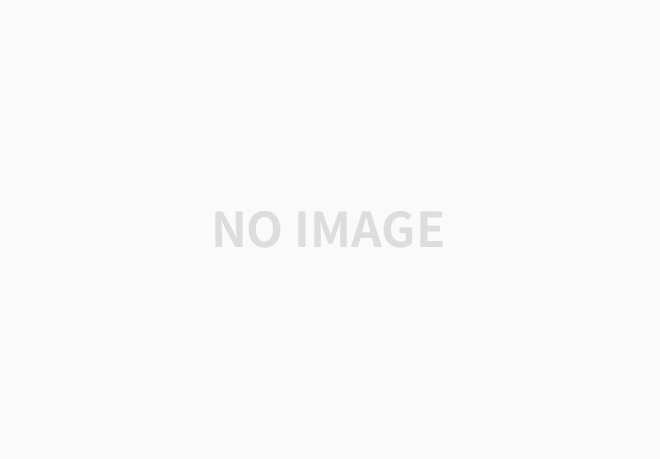
[urls.py]
from django.urls import path
from django.conf import settings
from . import views
urlpatterns = [
path('', views.upload_files, name='upload_files'),
path('upload', views.upload_files, name='upload_files'),
]
7. ajaxfilesupload APP의 views.py 설정
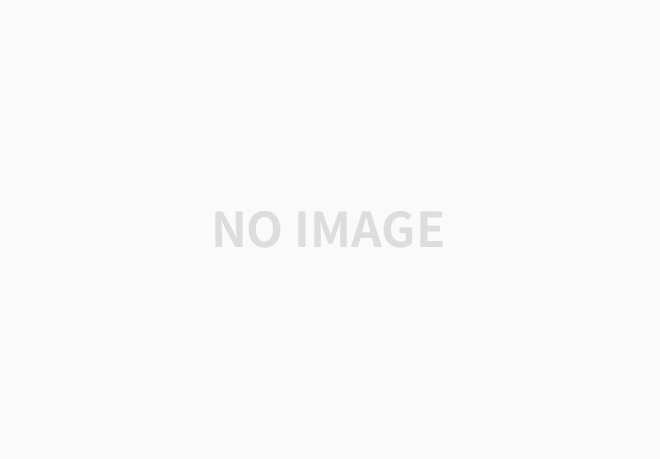
[views.py]
from django.shortcuts import render
from django.http import JsonResponse
from django.views.decorators.csrf import ensure_csrf_cookie
@ensure_csrf_cookie
def upload_files(request):
if request.method == "GET":
print('hi')
return render(request, 'files_upload.html', )
if request.method == 'POST':
files = request.FILES.getlist('files[]', None)
#print(files)
for f in files:
handle_uploaded_file(f)
return JsonResponse({'msg':'<span style="color: green;">File successfully uploaded</span>'})
else:
return render(request, 'files_upload.html', )
def handle_uploaded_file(f):
with open(f.name, 'wb+') as destination:
for chunk in f.chunks():
destination.write(chunk)
8. 실행 및 확인
[실행]
python manage.py runserver 0.0.0.0:8080
[확인]
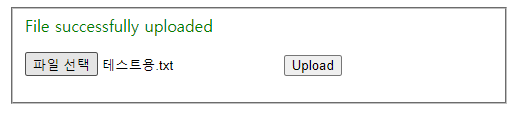
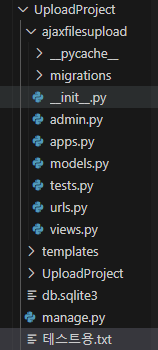
감사합니다.
'프로그래밍 > Django' 카테고리의 다른 글
[Django] 10. Custom User Model 구현( Serializer, Adapter) (721) | 2021.01.15 |
---|---|
[Django] 9. 로그인, 로그아웃 API 구현(JWT Token, RestFrameWork) (733) | 2021.01.14 |
[Django] 8. Mariadb / Mysql DB 연동(데이터베이스) (717) | 2020.12.23 |
[Django] 7. 파라미터 전송(정규표현식, URL 페이지 번호) (711) | 2020.12.16 |
[Django] 6. 파라미터 전송(GET, POST) (714) | 2020.12.15 |